First Time Experience Authoring a Kotlin DSL
I recently came across a file commented with // TODO convert to DSL
. I use this file frequently and while I thought it could be improved, it had never occurred to me that a DSL would help. Feeling curious and motivated by holiday down time, I attempted the task. I have no experience writing DSLs, but I have used them in various contexts—Gradle Kotlin DSL and the like.
This is certainly no replacement for the already great guidance available today. I used each of the following resources.
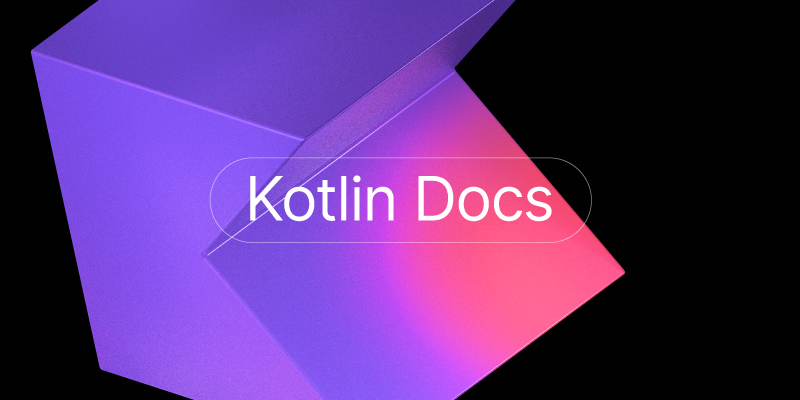

This is more a first timer's experience understanding code issues that a DSL would remedy and what to consider when constructing the DSL API.
Copy of a
A strong signal that a model may benefit from a DSL is heavy use of copy semantics. If you see call sites where an object is copied, cleared, and then edited to satisfy the use case, then perhaps it may help to evaluate whether developers find it too difficult to create the object.
val WORKING_MODEL = createModel(...)
// If a developer needs to copy a working model
// then perhaps they are not confident they
// can easily create one of their own
val ANOTHER_MODEL = WORKING_MODEL.copy(
someProperty = null,
otherProperties = emptyList()
)
Copying models is only as effective as the copy function itself. If a developer can easily copy an object, edit some properties, and then find themselves working with an invalid object such as a model with incorrect aggregate totals, then perhaps copying is not working well. Copying is not necessarily a bad thing, but the practice may indicate that creating the object is too difficult or cumbersome.
Builders All the Way Down
The Java Builder Pattern remains a perfectly fine pattern today, but if your objects or maybe a legacy library's models contain dozens of nested builders, then boilerplate may cause eyes to gloss over. Introducing a Kotlin DSL over-top existing builders improves the ergonomics of constructing these objects.
DSL Ergonomics
If you have identified that your component would benefit from a DSL, then consider the following API principles.
Defaults
Every domain is different, but defaults always matter. If your DSL is for the testing domain, then perhaps defaulting a model's ID is fine for all instances. If your model includes a quantity
then, default to 1 for the 90% of call sites.
Call Site Ordering
This seemed inherently obvious to me as a DSL user, but as an author, I was surprised how easy it is to forget about call site ordering. Take the following example.
recipe {
name("chili")
ingredient("pepper")
ingredient("salt")
}
// This should produce the same recipe
recipe {
name("chili")
ingredient("salt")
ingredient("pepper")
}
In this example, the call site order should not impact the resulting recipe. However, say you are assembling a cookbook where recipes are grouped by a category.
cookbook {
categories {
category(label = "breakfast", description = "...")
category(label = "dinner", description = "...")
}
recipes {
recipe(name = "scrambled eggs", category = "breakfast")
}
}
In this example, it is possible to require developers to declare all of the categories before all recipes. But simply delaying the grouping of recipes by category until after all the DSL entry points have been called enables developers to construct the object with any call site ordering.
Naming
Life is too short, just prefer functions for all DSL entry points instead of using Kotlin property syntax.
recipe {
// Just use functions. It's consistent with all DSL entry points
// ❌ name = "chili"
name("chili")
}
Fail on Build
Your object may be complex, and developers may inadvertently create invalid objects. In this case, fail to create the object and notify the developer of their error.
cookbook {
categories {
category(label = "breakfast", description = "...")
}
recipes {
// error with undefined category "snack"
recipe(name = "trailmix", category = "snack")
}
}
I was pleased with my first DSL, as it demonstrably improved both the authoring experience and readability for future maintainers. A DSL may feel more like a nice-to-have, which may be true, but I found that developers appreciate the improved ergonomics and most LLMs can bootstrap the initial API pretty well to cut down on dev time cost.